import tensorflow as tf ## 다운로드 from tensorflow.examples.tutorials.mnist import input_data mnist = input_data.read_data_sets("/AI/tmp/data/", one_hot=True) ## cnn 모델을 정의/;///; ## 3차원으로 reshape x_image = tf.reshape(x, [-1, 28, 28, 1]) ## 1번째 컨볼루션층 W_conv1 = tf.Variable(tf.truncated_normal(shape=[5,5,1,32], stddev=5e-2)) b_conv1 = tf.Variable(tf.constant(0.1, shape=[32])) h_conv1 = tf.nn.relu(tf.nn.conv2d(x_image, W_conv1, strides=[1,1,1,1], padding='SAME')+ b_conv1) ## 1번쨰 풀링층 h_pool1 = tf.nn.max_pool(h_conv1, ksize=[1,2,2,1], strides=[1,2,2,1], padding='SAME') ## 2번째 컨볼루션층 W_conv2 = tf.Variable(tf.truncated_normal(shape=[5,5,32,64], stddev=5e-2)) b_conv2 = tf.Variable(tf.constant(0.1, shape=[64])) h_conv2 = tf.nn.relu(tf.nn.conv2d(h_pool1, W_conv2, strides=[1,1,1,1], padding='SAME') + b_conv2) ## 2번째 풀링층 h_pool2 = tf.nn.max_pool(h_conv2, ksize=[1,2,2,1], strides=[1,2,2,1], padding='SAME') ## 완전 연결층 W_fc1 = tf.Variable(tf.truncated_normal(shape=[7*7*64, 1024], stddev=5e-2)) b_fc1 = tf.Variable(tf.constant(0.1, shape=[1024])) h_pool2_flat = tf.reshape(h_pool2, [-1, 7*7*64]) h_fc1 = tf.nn.relu(tf.matmul(h_pool2_flat, W_fc1) + b_fc1) ## 출력층 W_output = tf.Variable(tf.truncated_normal(shape=[1024, 10], stddev=5e-2)) b_output = tf.Variable(tf.constant(0.1, shape=[10])) logits = tf.matmul(h_fc1, W_output) + b_output y_pred = tf.nn.softmax(logits) return y_pred, logits ## 인풋, 아웃풋 데이터 받기위한 정의 x = tf.placeholder(tf.float32, shape=[None, 784]) y = tf.placeholder(tf.float32, shape=[None, 10]) ## Convolutional Neural Networks(CNN) 선언 y_pred, logits = build_CNN_classifier(x) ## 손실함수와 옵티마이저 정의 loss = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(labels=y, logits=logits)) train_step = tf.train.AdamOptimizer(1e-4).minimize(loss) ## 정확도 계산 연산 correct_prediction = tf.equal(tf.argmax(y_pred,1), tf.argmax(y,1)) accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32)) ## 세션열어 학습진행 with tf.Session() as sess : sess.run(tf.global_variables_initializer()) ## 1000만큼 최적화 수행 for i in range(10000) : ## 50 개씩 mnist 데이터 불러오기 batch = mnist.train.next_batch(50) ## 100개마다 training 데이터셋의 정확도 출력 if i % 100 == 0: train_accuracy = accuracy.eval(feed_dict={x:batch[0], y:batch[1]}) print("반복(epoch) : %d, 트레이닝 데이터 정확도 : %f" % (i, train_accuracy)) ## 옵티마이저를 실행해 파라미터를 한 스텝 업데이트 sess.run([train_step], feed_dict={x:batch[0], y:batch[1]}) ## 학습이 끝나면 테스트 데이터에 대한 정확도 출력 print("테스트 데이터 정확도 : %f" % accuracy.eval(feed_dict={x:mnist.test.images, y:mnist.test.labels}))
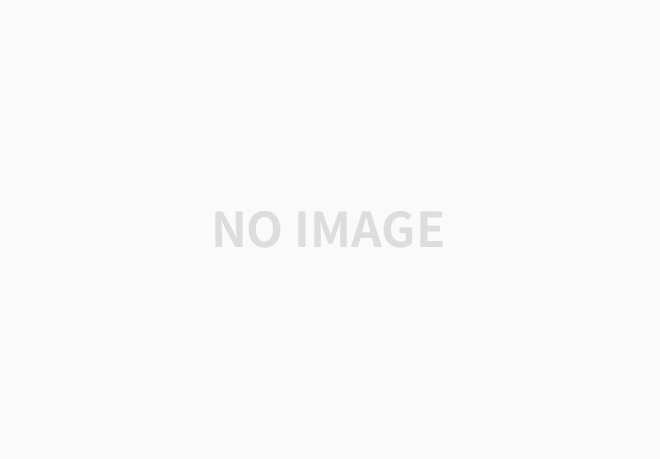
> tf.train.Saver API를 이용해서 모델과 파라미터를 저장하고 불러오기
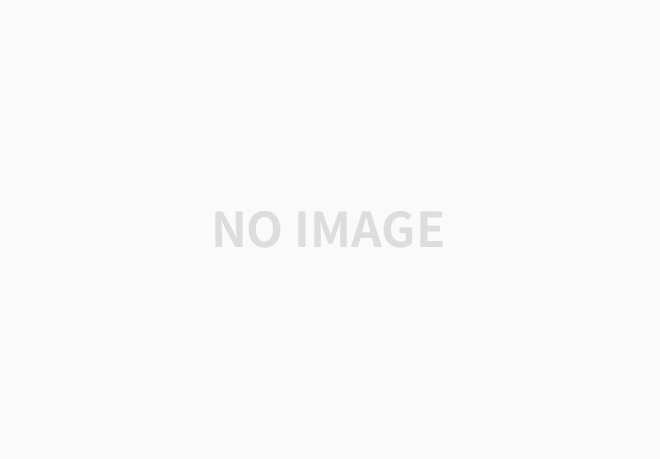
+ 추가된 부분
## 정확도 계산 연산 correct_prediction = tf.equal(tf.argmax(y_pred,1), tf.argmax(y,1)) accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32)) ## tf.train.Saver를 이용해서 모델과 파라미터를 저장 SAVER_DIR = "model" saver = tf.train.Saver() checkpoint_path = os.path.join(SAVER_DIR, "model") ckpt = tf.train.get_checkpoint_state(SAVER_DIR) ## 세션열어 학습진행 with tf.Session() as sess : sess.run(tf.global_variables_initializer()) ## 만약에 저장된 모델과 파라미터가 있으면 이를 불러오고 (Restore) ## restored 모델을 이용해서 테스트 데이터에 대한 정확도를 출력하고 프로그램 종료 if ckpt and ckpt.model_checkpoint_path: saver.restore(sess, ckpt.model_checkpoint_path) print("테스트 데이터 정확도(Restored) : %f" % accuracy.eval(feed_dict={ x:mnist.test.images, y:mnist.test.labels})) sess.close() exit()
## 100개마다 training 데이터셋의 정확도 출력 if i % 100 == 0: saver.save(sess, checkpoint_path, global_step = i)
+) 피피티에는 빠져있는데 반드시 모델 save 해주어야함!
코랩 참고 사이트
https://jisoo-coding.tistory.com/2
https://tykimos.github.io/2019/01/22/colab_getting_started/
'딥러닝' 카테고리의 다른 글
0814 embedding으로 단어 연관성 예측하기 (0) | 2019.08.14 |
---|---|
0813 ResNet 프로젝트 (0) | 2019.08.13 |
0812 ANN을 이용한 MNIST 숫자 분류기 구현 (0) | 2019.08.12 |
0812 소프트맥스 회귀로 MNIST 데이터 분석하기 (0) | 2019.08.12 |
0812 텐서플로 기초 (0) | 2019.08.12 |